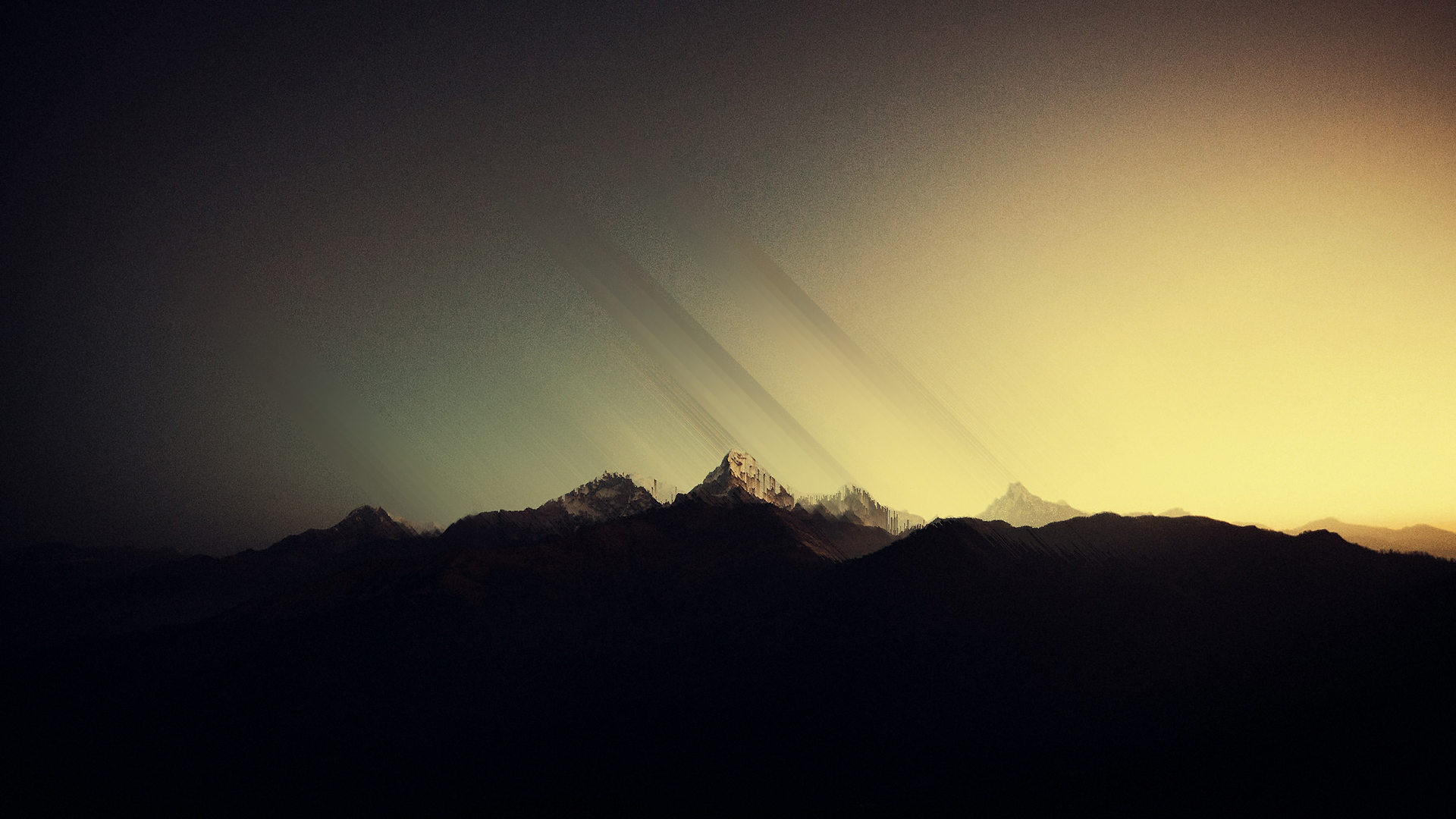
// Game Programmer

QORE ENGINE
ABOUT
Company
Primary Role(s)
Game Engine
Project Status
Project Type
Languages
​Independent
Software Engineer
Qore
Released
Personal
C++, Lua
The Qore Engine is a 2D game engine built using C++ and Lua, with the SDL and GLM libraries.
My Contributions :
-
Built the architecture of a 2D game engine from the ground up.
-
Used C++ as a core language and Lua for embedded scripting and configuration.
-
Utilized SDL library to handle graphics, sound, and hardware input.
-
Wrote a game loop that involves initializing entities into the game world, handling update cycles with a fixed time step, and rendering visual & auditory elements.
-
Created a component-based system that allows for attachable modules such as sprite, transform, collider, sound, text, and emitter components.
---
Building Qore was an incredibly enlightening experience. After having done so, I can firmly say that every game programmer should try their hand at building an engine of their own. Initially, I had many questions regarding Unity and UE4. Not only were those questions answered after building Qore, but I was able to apply my newfound knowledge back into those game engines to improve my work flow. As a result, I now feel comfortable adopting totally new game engines as I now understand the base mechanics behind them.
Every game engine contains a core loop that contains processes and events dictating what can happen within a single frame. The loop I used contained:
1: ProcessInput(), which observed what keys the user held up or down at any given frame.
2: Update(), which is responsible for calculating ticks (which I clamped to run at 60 FPS), and deltaTime.
3: Render(), which actually draws sprites, tilemaps, and UI to the screen. I utilized a double buffering technique for graphics display.
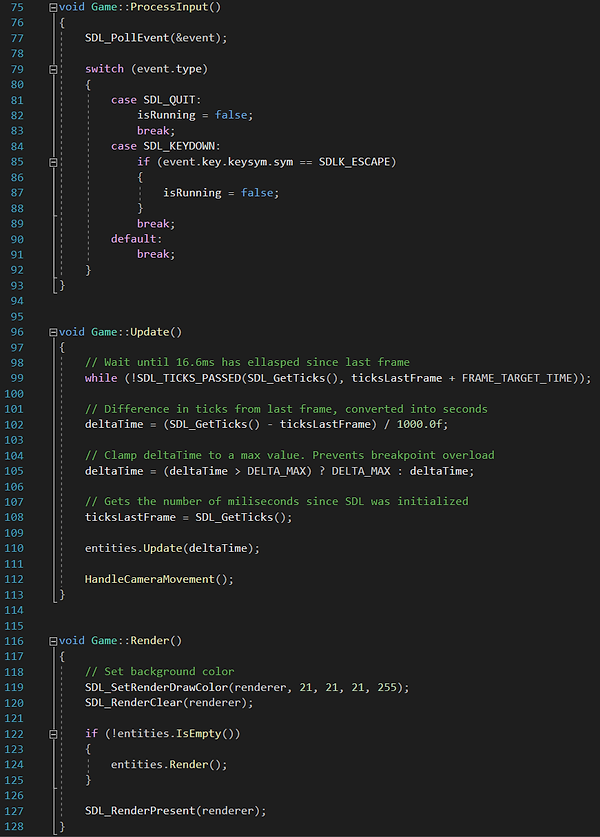
A key idea to a game loop, especially as it relates to a game engine, is a cascading design. I opted to pass methods inside the core game loop down to each entity in the game, so that each object can successfully update their state each tick. For instance, checking collision is made easier now that the entity's transform is being updated every tick. Within each entity component, these central methods are passed down so that every object in the game can coordinate with each other inside the same frame.

There are a couple helper classes that help organize all my entities. The first being the AssetHandler. This class is responsible for handling all assets, whether that be textures or fonts. The engine can then call upon the class to display a sprite or label onto the screen.
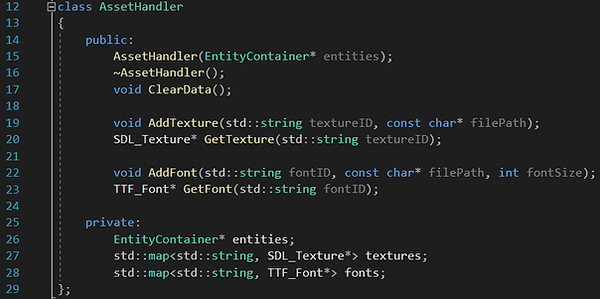
Next, we have the EntityContainer. This class is responsible for holding all the entities in the current game state. This is essential, as the core game loop needs to iterate every object to update or render in the game before 1 frame.

Further down the line, we have the actual Entity class. I utilized template methods for adding/getting components, so that I could consolidate all component types into one class. An entity is essentially any "thing" that exists inside a game. This could be a texture, sound, label, etc...
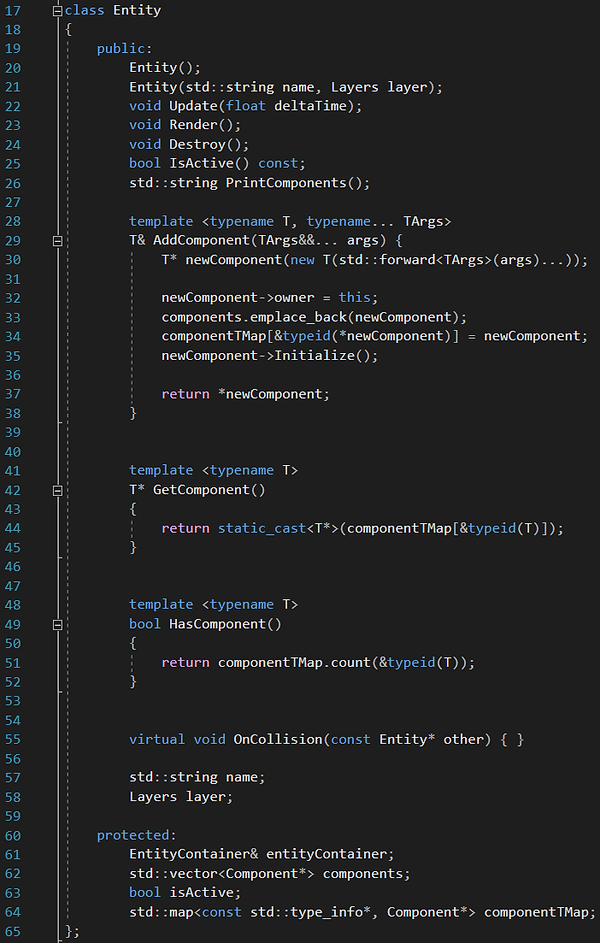
Lastly, the base Component class does practically nothing, but allows more specific component classes to inherit the Update and Render methods.

I opted for a component based system, much like Unity, to handle what an entity is capable of in-game. Writing it this way allows for easy modularization. Developers working with Qore can easily write a Lua script to add, remove, or get all sorts of components at any time.
​
The first example, and one of the essential components, is the TransformComponent. This overrides the Update method and uses the GLM library to position, in x & y pixel coordinates, the exact location of an entity on the screen. Note, that this doesn't actually draw anything (that is handled by the SpriteComponent).
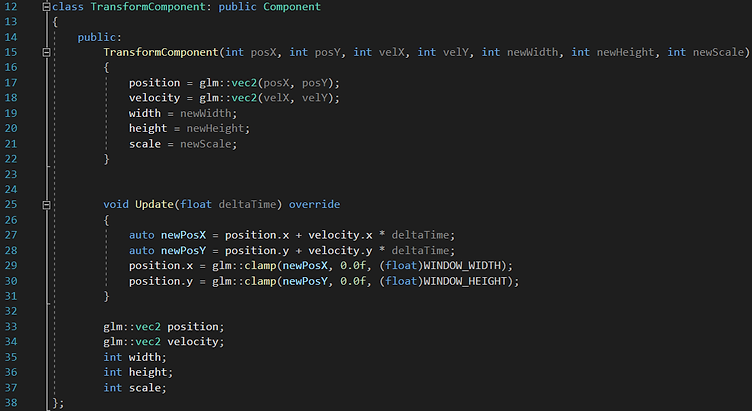
Displaying UI text on the screen is similar to displaying a sprite. However, I needed to make use of a font family. The component class I wrote below shows how I used SDL to create surface. A surface allows for fonts to be displayed. I then created a texture from that surface to be used for drawing on screen.

The last component I'd like to take a look at is the AudioComponent. I utilized the SDL_Mixer class to help with initializing and playing audio. I allowed for looping and playing on awake for ease of use.
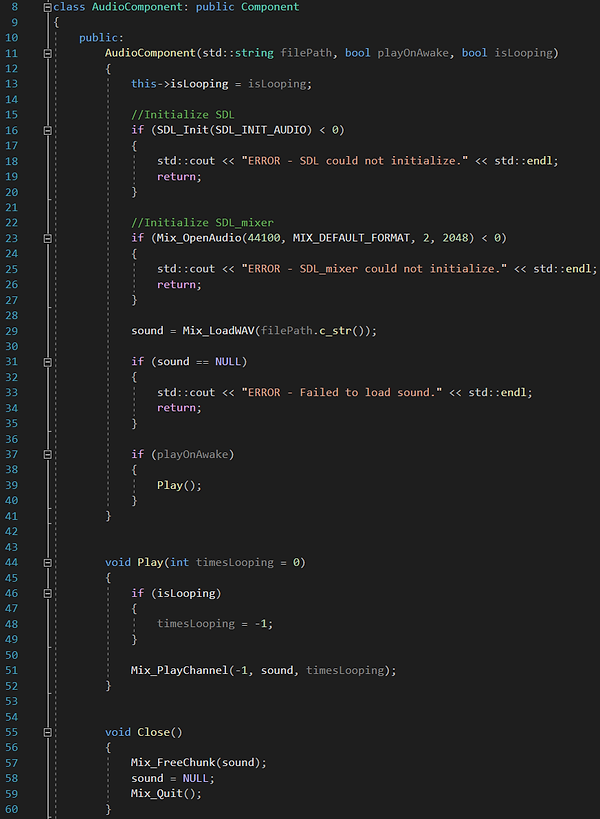